Description
Represents positions, rotations, size, 3D vectors, and points. Contains functions for doing common vector operations.
Properties
magnitude |
The length of this vector (Read Only). |
normalized |
The vector sized to a magnitude of 1 (Read Only). |
x |
X component of the vector. |
y |
Y component of the vector. |
z |
Z component of the vector. |
Methods
angle |
Finds the angle between this vector and another (degrees). |
cross |
Finds the cross product of this vector and another. |
dot |
Finds the dot product of this vector and another. |
lerp |
Linearly interpolates between two vectors. |
project |
Transforms the vector from one coordinate space to another. |
Constructor
Vec3 |
Constructs a vector by xyz component values. |
Operators
- |
Subtracts one vector from another. |
!= |
Compares two vectors. |
* |
Multiplies a vector by another vector or a scale factor. |
/ |
Divides a vector by another vector or a scale factor. |
+ |
Adds two vectors together. |
+= |
Assigns the vector to be the sum of two vectors. |
-= |
Assigns the vector to be the difference of two vectors. |
== |
Compares two vectors. |
unary - |
Negates the vector. |
Details
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.magnitude
readonly double magnitude
Description
The length of this vector (Read Only).
The length of the vector is calculated as the square root of (x*x+y*y+z*z).
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.normalized
readonly Vec3 normalized
Description
The vector sized to a magnitude of 1 (Read Only).
A copy of the original vector that points in the same direction, but has a length of 1.
Do no remove, this fixes the anchor on doc.flexsim.com
double x
Description
X component of the vector.
Do no remove, this fixes the anchor on doc.flexsim.com
double y
Description
Y component of the vector.
Do no remove, this fixes the anchor on doc.flexsim.com
double z
Description
Z component of the vector.
Do no remove, this fixes the anchor on doc.flexsim.com
Parameters
otherVec |
The angle extends round to this vector. |
Returns
double
|
The angle between the two vectors in degrees. |
Description
Finds the angle between this vector and another (degrees).
double angle = myVector.angle(Vec3(1, 2, 3));
Do no remove, this fixes the anchor on doc.flexsim.com
Parameters
otherVec |
The vector to cross with this vector. |
Returns
Vec3
|
The cross product (Vec3) of the two vectors. |
Description
Finds the cross product of this vector and another.
Vec3 cross = myVector.cross(Vec3(1, 2, 3));
The cross product of two vectors is a vector perpendicular to the two input vectors. Since there are two possible perpendicular vectors, the direction is determined by the "left hand rule". This means that crossing vector1 with vector2 will
produce the opposite of crossing vector2 with vector1. The magnitude of the new vector is equal to the magnitudes of the two inputs multiplied together and then multiplied by the sine of the angle between the inputs.
Do no remove, this fixes the anchor on doc.flexsim.com
Parameters
otherVec |
The vector to dot with this vector. |
Returns
double
|
The dot product (double) of the two vectors. |
Description
Finds the dot product of this vector and another.
double dot = myVector.dot(Vec3(1, 2, 3));
The dot product of two vectors is a number equal to the magnitudes of the two vectors multiplied together and then multiplied by the cosine of the angle between them.
Do no remove, this fixes the anchor on doc.flexsim.com
Parameters
otherVec |
The vector to interpolate to. |
t |
A ratio between 0 and 1 that defines the percentage to interpolate from the current vector to otherVec. |
Returns
Vec3
|
The resulting interpolated vector. |
Description
Linearly interpolates between two vectors.
The following example code changes current's location 20% towards the other object.
current.location = current.location.lerp(otherObj.location, 0.2);
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.project()
Parameters
from |
The treenode representing the coordinate space to project from. |
to |
The treenode representing the coordinate space to project to. |
Returns
Vec3
|
The projected vector. |
Description
Transforms the vector from one coordinate space to another.
Takes the vector in from's coordinate space, translates this vector onto to's coordinate space, and returns the projected vector.
The vector is taken from the origin point of from's coordinate space. The origin is the (0,0,0) point of the coordinate space and is often located in the top left corner of an object's coordinate space.
It is important to understand the way the axes work in FlexSim. The X and Y axes form the floor grid plane while the Z axis is equivalent to the height. Also objects y size values stretch in the
-y direction, but stretch in the positive direction on both the X and Z axes. In other words an object at location (0,5,0) with a size of (1,2,3) will stretch from x location 0 to 1, y location 5 to 3 and z location 0 to 3.
Vec3 globalLoc = Vec3(5, 3, 0).project(current, model());
This example finds out the global location of the position (5,3,0) in current's coordinate space.
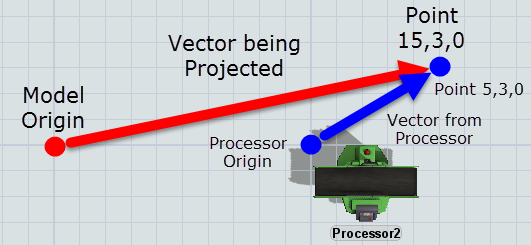
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3 Constructor
Parameters
x |
The x component. |
y |
The y component. |
z |
The z component. |
value |
A value all components will be set to. |
Description
Constructs a vector by xyz component values.
current.location = Vec3(1, 2, 3);
current.location = Vec3(0);
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.operator -
Parameters
otherVec |
The vector to subtract from this one. |
Returns
Vec3
|
The resulting vector. |
Description
Subtracts one vector from another.
Each component has the corresponding component subtracted from it.
current.location = someVector - anotherVector;
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.operator !=
Parameters
otherVec |
The vector to compare to this vector. |
Returns
int
|
True if the two vectors are different, false otherwise. |
Description
Compares two vectors.
int isNotZero = current.location != Vec3(0, 0, 0);
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.operator *
Parameters
otherVec |
The vector to multiply this vector by. |
factor |
A scale factor to multiply each component by. |
Returns
Vec3
|
The resulting vector. |
Description
Multiplies a vector by another vector or a scale factor.
When a Vec3 is passed, the operation will perform per-component multiplication.
current.location = someVector * anotherVector;
current.location = someVector * 1.2;
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.operator /
Parameters
otherVec |
The vector to divide this vector by. |
factor |
A scale factor to divide each component by. |
Returns
Vec3
|
The resulting vector. |
Description
Divides a vector by another vector or a scale factor.
When a Vec3 is passed, the operation will perform per-component division.
current.location = someVector / anotherVector;
current.location = someVector / 1.2;
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.operator +
Parameters
otherVec |
The vector to add to this one. |
Returns
Vec3
|
The resulting vector. |
Description
Adds two vectors together.
Each component is added to the corresponding component.
current.location = someVector + anotherVector;
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.operator +=
Parameters
otherVec |
The vector to add to this one. |
Returns
Vec3
|
The resulting vector. |
Description
Assigns the vector to be the sum of two vectors.
Each component is added to the corresponding component.
current.location += someVector;
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.operator -=
Parameters
otherVec |
The vector to subtract from this one. |
Returns
Vec3
|
The resulting vector. |
Description
Assigns the vector to be the difference of two vectors.
Each component has the corresponding component subtracted from it.
current.location -= someVector;
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.operator ==
Parameters
otherVec |
The vector to compare to this vector. |
Returns
int
|
True if both vectors are exactly the same, false otherwise. |
Description
Compares two vectors.
int isZero = current.location == Vec3(0, 0, 0);
Do no remove, this fixes the anchor on doc.flexsim.com
Vec3.operator unary -
Returns
Vec3
|
The resulting vector. |
Description
Negates the vector.
Each component is negated.
current.location = -current.location;